27 Oct 2022 - Activity - Deconstructing Waves
Contents
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
27 Oct 2022 - Activity - Deconstructing Waves#
While we observe waves in many places, it’s the case that we don’t often have the luxury of constructing them as we want. In fact, much of the science we do relies on measurements of voltage or current to represent the dynamics of the system.
Want to measure distance? Interferometry gives you a voltage to calibrate.
Want to measure material surface properties? Voltage jumps across probes give you proxies
Viscosity? Stick the stuff in a rheometer, squeeze it, and measure voltage changes.
AYO exoplanets? Light curves are really just measures of voltage across a CCD.
The point is that you will almost always be dealing with signals that are proxies for the actual thing you care about. So let’s construct some and deconstruct them.
Below is the code that we developed last class to investigate the signals we produced.
t = np.linspace(0,2,1000)
omega1 = 100
omega2 = 105
omega3 = 1000
A1 = 1
A2 = 0.9
A3 = 0.1
y1 = A1*np.cos(omega1*t)
y2 = A2*np.cos(omega2*t)
y3 = A2*np.cos(omega3*t)
y4 = A3*np.cos(omega2*t)
y5 = A3*np.cos(omega3*t)
CloseAmpCloseFreq = y1+y2
CloseAmpFarFreq = y1+y3
FarAmpCloseFreq = y1+y4
FarAmpFarFreq = y1+y5
fig = plt.figure(figsize=(16,8))
plt.plot(t,CloseAmpCloseFreq+6, label='Close Amp; Close Freq')
plt.plot(t,CloseAmpFarFreq+2, label='Close Amp; Far Freq')
plt.plot(t,FarAmpCloseFreq-2, label='Far Amp; Close Freq')
plt.plot(t,FarAmpFarFreq-6, label='Far Amp; Far Freq')
plt.legend()
<matplotlib.legend.Legend at 0x1193d7640>
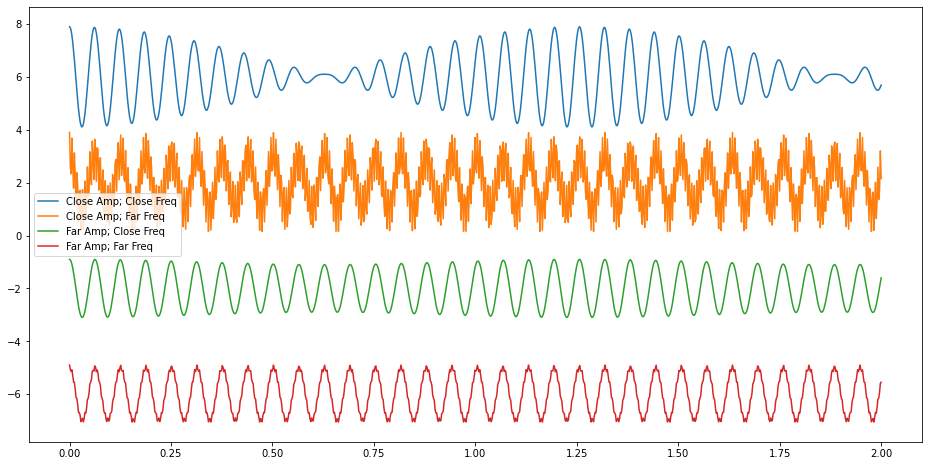
Questions:#
Adjust the signals to show qualitatively how the different signals behave as you change frequencies and amplitudes.
Build a table that discusses qualitatively what happens as each change is made. We will compile this table together.
Dealing with “Real” Signals#
Ok, but can we find these frequencies given a signal? Or rather, how might we find the signal we need? We can use a little mathematics from Fourier.
Any periodic function in 1 dimension can be expanded as a general sum of sines and cosines:
We can show this can be written like:
But how does this get us what we need? Our goal is to find the expansion coefficients (
from scipy.signal import sawtooth, square, chirp
t = np.linspace(0,10,1000)
saw = sawtooth(t)
sq = square(t)
ch = chirp(t,6,10,1)
fig = plt.figure(figsize=(12,8))
plt.plot(t, saw, label="Sawtooth Wave")
plt.plot(t, sq+3, label='Square Wave')
plt.plot(t, ch-3, label='Chirp')
plt.legend()
<matplotlib.legend.Legend at 0x16ce735b0>
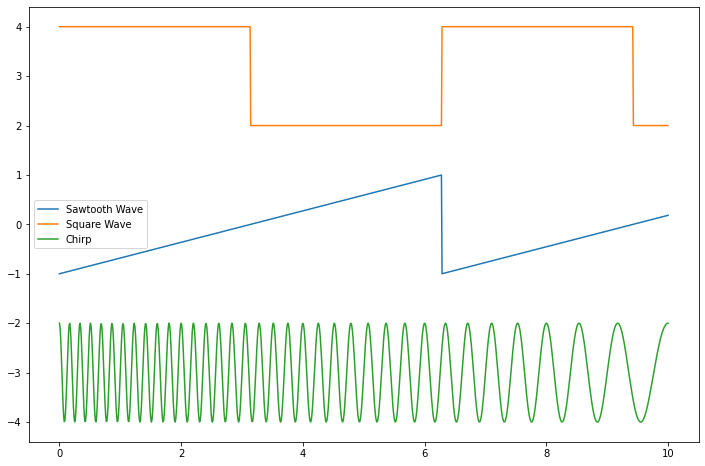
Integration Activity#
Consider the Fourier Expansion of your choosing:
In terms of the longest period,
Find the expansion coefficients for the following signals (think before you integrate):
here corresponds to the longest known period . from 0 to and 0 from to repeating….
1D Integration#
It’s not great doing this by hand. Certainly, we can pick off the first two easily because they match our model easily. What about the 3rd one?
It’s clever to change this to a cosine and read off the values:
And thus:
Can we do the integrals and get the same results? Let’s form the integrals we need to do this. The left hand side uses the real function
Integrals that solve this problem#
We start with the sine function first:
Notice we get three integrals on the right side (where we moved the integral inside the sum as we can do and focus on the
The left side uses the real
Thus, all the
More integrals that solve this problem#
Now the cosine function:
Notice we get three integrals on the right side (where we moved the integral inside the sum as we can do and focus on the
The left side uses the real